Methods To Integrate Application Components in the Application |
|
The
application may comprise of a collection of components
(e.g. AC). These components may need to communicate
with each other. The communication can involve either
simple data passing or it could involve two or more
components coordinating some activity. Wherein, some
means of connecting the components to each other is
needed. |
|
Usually
the ACs in the web page (or in the application) need
to communicate with each other (e.g. exchange data or
one AC notifies an event to another AC, such as, mouse
clicks, state-change or data arrival.). To accomplish
this the AC must be integrated with other components. |
|
For
example, one or more ACs may be created by CCG (e.g.
CF or GUI-CCG), and their code is copied in to the web-document,
as shown in the fig#1 and Fig#2. Some of the preferred
methods to integrate the ACs in the web-document are
given below. |
|
A.
Using Integration Logic at the server to generated code
to integrate the ACs: |
|
Many
Application components in the applications usually need
to communicate with one or more other ACs or external
code. If Java Classes (e.g. CF or GUI-CCG) generate
the ACs, the ACs need to be integrated, so that, they
can exchange data or communicate with each other. The
following figure illustrates the process to include
the integration code in the web document, which facilitates
inter component communications. |
|
Fig#1 |
|
The
figure#1 shows that a container-CF uses three Java objects
(e.g. CF or GUI-CCG) to generate its subcomponents.
The CF further contain Java code (which is referred
to as Integration-Logic or “IL”), which
interacts with the sub-CCG to get names of service functions
of the ACs or to set callbacks for the ACs; and uses
these functions to generate integration code. Therefore,
the code for the container-AC comprises of the code
for the sub-ACs and their integration code. |
|
Each GUI Component is placed at Proper Location |
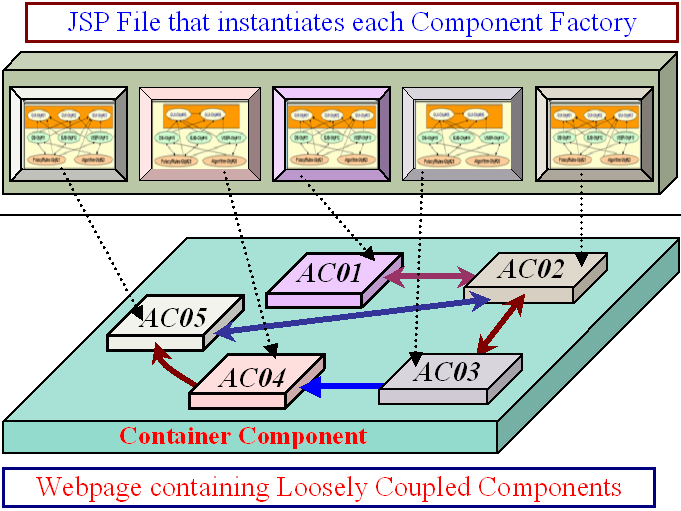
|
Arrows
Represent Loosely Coupled Service Interfaces
If the Loosely Coupled Components (e.g. AC01 to AC05) need to collaborate with other,
they Interact with each other by requesting each other services at run-time.
|
New
kind of perfect Loosely Coupled Components
(Click on above to learn about
the Component Making/Packaging)
It requires just 2 lines to include each
CF/AC (Click here for
example). If
any two ACs needs to collaborate with each other, on average they need 3 to 5
more lines of communication code (e.g. to loosely couple service-provider and service-consumer
ACs).
|
|
|
Likewise,
the JSP or Servlet may use one or more CCG-Objects to
include their ACs in the web-document. Furthermore,
the JSP may also generate necessary integration code
and include it in the web-document. |
|
|
Fig#2a(Above)
&
2b(Below)
|
|
Fig#2c(Below) |
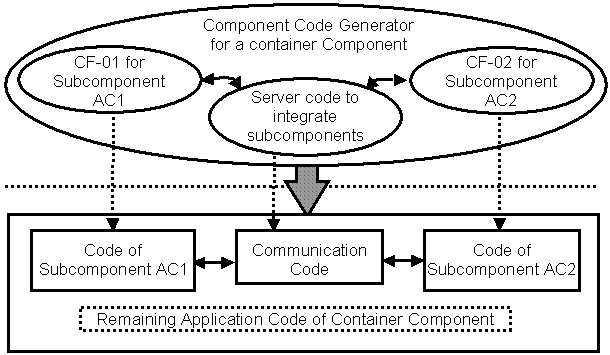
|
|
Note:
Upon an event on AC2, the AC2 calls the callback
(Blue-dotter-arrow
in Fig#2b). The callback calls the service method of AC1 (Red-dotter-arrow
in fig#2b)
|
|
The
figure#2 above and listing#1 below shows a simple method
to integrate two ACs that are generated by the CCGs.
The JSP (or container CCG) instantiates and initializes
two CCGs, for example, CCG1 and CCG2. The code uses
their CGM to get the code for the subcomponents AC1
and AC2. It places the code for the subcomponents and
their integration code at appropriate location in its
component code. The following pseudo code illustrates
a sample “integration logic” that generates necessary code for the
two ACs to communicate with each other. |
|
Pseudo-code
listing#1 |
Function CGM( out, req) {
// Code Generation Method for the Container-CCG
// Instantiate and
Initialize the CCG1
CCG1.CGM(Out, req);
// Call Code Generation
Method
// It writes the
code for the AC1-code to OUT stream
// Integration logic to integrate the components.
// Get the name of the AC1’s service method.
String ac1_service_method
= CCG1.getServiceMethodName();
// Generate integration
code. This function calls the service method
of CCG1
Out.println(“ function CB_of_AC2_gets_service_of_AC1
( par1, par2) { “);
Out.println(“ “ + ac1_service_method
+ “(par2);” );
Out.println(“} “);
// Instantiate and
Initialize the CCG2
// Register the callback. Upon given event,
the AC2 calls the callback
CCG2. setCB_AddMethodName(“CB_of_AC2_gets_service_of_AC1”);
// The above
statement makes the CGM to create the Subcomponent
code
// So that, it call the given JavaScript function
upon certain event.
CCG2.CGM(Out, req); //
Call Code Generation Method
// It writes
the code for the AC2-code to OUT stream
} |
|
|
The
pseudo code shows a method to integrate two subcomponents,
such that, one subcomponent AC2 gets a service of the
other subcomponent AC1. To accomplish this, the container-CCG
comprise of code that interact with the CCG-objects
to get necessary data about the subcomponents (i.e.
AC1 and AC2) and to generate the integration code. The
code (referred to as integration logic) calls the service
method of CCG1 to get the name of the service method
of the subcomponent AC1. The integration logic also
generates body of a callback function (e.g. CB_of_AC2_gets_service_of_AC1),
which may be used to call the service method of AC1.
Then the integration logic calls the service method
of CCG2 to register the callback name for the subcomponent
AC2. This statement causes the CCG2 to generate the
AC2 code, such that, the AC2 calls the callback-function
upon the given event (e.g. mouse click) or a change
in the state in the subcomponent AC2. When the callback
function is run, it calls the AC1's service method. |
|
B.
Using Directory services to access services of other
ACs: |
|
The
ACs in the application (or web document) may communicate
by employing publish and subscribe architecture. Refer
to following figure. Publish and subscribe system comprises
of a Registration-Component (or Object) to hold Directory
of services. The name of the Registration-Component
may be reserved word or predefined and its interfaces
(or methods) are published in advance (e.g. documented
and shared with teams at the early stages of the design
of the application). Therefore, the CF or CCG may be
designed to generate its AC’s code, such that,
the AC registers the services that it offer. Likewise,
other AC may also lookup for the services that it needs. |
|
As
shown in the figure#3, each AC in the web document
may register their services with the Registration Component.
To register each service, the AC calls the Registration-Component’s
method to input a lookup-name for the service and reference
to the method (or Object) to get the service. Any AC
in the application may get the service by using the
lookup-name for the service. |
|
For
example, in a simple shopping-cart application where,
upon selection to buy, each shopping-item needs to notify
its data (e.g. its price, model and other data) to the
Invoice/Table component, so that, the item could be
added to the Invoice’s Table. To accomplish this,
the Invoice’s AC could register its service using
a unique service-name (e.g. ShoppingCart); and upon
selection each shopping item’s AC lookup for the
service using the name “ShoppingCart” to
request the service. The service-name (e.g. ShoppingCart)
and data format can be predefined. |
|
Example:
Use of registration class |
|
The
SVG document may have global variables and objects.
It is possible to define a service registration class
(e.g., 'service_register_class') and instantiate a global
object with a reserved name such as 'service_register_obj'.
Now, any AC can access this object using this reserved
name (one version of service registration class is disclosed
in Appendix A). Multiple components or subcomponent
class objects may be registered according to the general
steps shown in FIG. 3. |
|
Fig#3 |
|
The
'service_register_class' can be implemented so that
it supports methods to register services with a service-name.
Also, service can be accessed by methods using the service-name
ID. Using this technique, any component can register
its service with a unique name. If any other component
needs that service, the component can look up the service
using the name. For example, at the beginning of the
SVG document, the service registration object may be
instantiated: |
|
var services_register_obj
= new service_register_class(); |
|
The
CCG object can generate code to register the AC’s
service with the 'service_register_object' using a unique
service-name. For example, the following statement may
be included in the AC’s code to add the AC’s
service: |
|
services_register_obj.add_service("ShoppingCart",
shopping_cart_service); |
|
If
the CCG classes that creates presentation code for the
other ACs know the service-name, they can include the
following instruction in the ACs to get the service: |
|
services_register_obj.get_service("ShoppingCart",
xml_data, callback_name); |
|
Developers
of both client components and server components may
agree on the names of the services in advance or may
use other mechanisms to determine the service-name. (Appendix B comprises of an example) |
|
C.
Accessing Services Of Predefined Utility AC: |
|
The
Application may comprise of one or more global ACs,
whose integration interfaces are predefined (e.g. at
the early stages of the design of the application).
The predefined interfaces may include, the AC’s
names and interfaces of the functions to get its services.
Usually the Global AC provides generic or common services,
such as, status-bar or information-box to display information
or help. Any AC in the application could get their services
to display status, help or information-messages. |
|
For
example, many GUI-applications, such as, Microsoft’s
Internet Explorer or “IE” comprised of “Status
Bar” that displays status and information, such
as, the URL if mouse moves onto a hypertext-link; or
progress, while down loading a web page. |
|
Any
AC in the application may get the services of such utility
ACs, if their names and interfaces defined and documented
in advance (e.g. at the beginning of the application
design). The CCG may be designed to use predefined names
and documented interfaces of the utility ACs, to build
its AC, such that, the AC could get the services. Alternatively
the CCG comprise of methods to input the information,
which may be used to generate the AC, so that, it can
communicate with other ACs. |
|
Appendix-A::
A Simple Service Registrstion Object |
|
Sample
Registration Class code |
//SECTION ONE: Class definition for Register Component
function service_register_class() {
var services_func = new Array(); // An array to save
References to Service-functions or Objects
var services_str =
new Array(); // An array to save Respective Names to lookup the services
// This function
registers or Adds a service and its name.
// The simple
implementation just adds them to respective arrays
this.add_service
= function(name, func) {
services_str[services_str.length]= name;
// Add the Service Name at next Index
services_func[services_func.length] =
func; // Add the Service-Function or Object
}
// This function
searches for the service function for "ser_str"
// If found, it
returns the reference to the service function
this.find_service
= function (ser_str) {
for(i = 0; i
< services_str.length; i++)
if(ser_str ==
services_str[i])
// When found the name
return (services_func[i])
//
Return the reference
return(null)
}
// This function
searches for the service function for "ser_str"
// If found, it
executes the function by passing the parameters.
this.get_service
= function (ser_str, par1, par2, par3) {
for(i = 0; i
< services_str.length; i++) {
if(services_str[i] == ser_str)
return ((services_func[i])(par1, par2, par3))
}
return(null)
}
} // End Of Class
definition
//SECTION TWO: Object Instantiation of Register Object
var service_register_obj = new
service_register_class();
// To add a servise: service_register_obj.add_service("Shopping
Cart1", add_to_invoice);
// To find a service: service_register_obj.find_service("Shopping
Cart1");
|
|
|
Note:
The appendix-B provides an example and appendix-C presentation
a basis for interface contracts between the “loosely
coupled” components in the application. You may
skip them for now and read when you like to learn about
the interface-contracts. |
|
Appendix-B::
Shopping Cart Example for the Application Components |
|
The
shopping cart applications usually contain two types
of components or ACs: |
|
1. |
A
Shopping-cart AC, which is usually an Invoice-table
(see figure#5). It supports one or more services, such
as, a function (or method) to add an item to the Cart
and another function to remove an item from the cart. |
|
2. |
Multiple
AC’s one for each item, one can shop. The shopping-items
may support one or more service methods. For example,
methods such as, a function to register a callback.
If user selects the item to purchase (e.g. by double
clicking on the item), the AC calls the callback and
passes the information about the item in one of the
parameters. This data may be passed in an Object or
an XML-string. The format of the Object or XML-string
is predefined by the Shopping-cart. This information
comprises of data pieces, such as, barcode, model, make,
volume-discounts, inventory and size etc. |
|
The
CF can be designed to hide the manufacturing process
of the AC. The AC may comprise of one or more sub-ACs.
For example, if the items are Cars: it may display a
sub-AC, which shows detailed information about the Car,
when mouse moves onto the AC. Upon one-click, the AC
may display another sub-AC, which contains form GUI-components,
such as, check boxes to select additional options (e.g.
Manual or Auto-transmission and Stereo or CD-player);
and drop down list to select the color. |
|
Furthermore,
the CF may be designed to use the information, such
as, user-profile (e.g. preferred customer), policies
(e.g. inventory clearance), algorithms (e.g. seasonal
dynamics, historical trends, or supply and demand forecast
mismatch) security (e.g. private data to the sales force,
distributors or VARS) and other dynamic business factors.
The CF manufactures the AC. |
|
The
CF, a Java-class, may be independently designed. Likewise,
the CF for the Invoice-table may be designed independently.
The CFs can generate the custom ACs. The code-block
for the ACs can be copied in to the web-document and
integration the ACs (figure#1 and #2), so that the Item’s
callback calls the Shopping-cart’s service method. |
|
The
AC can be integrated using any method, such as, Integration-logic
or Directory-services method. If the components use
Directory-services, the CF may get the name of the service
as one of the inputs as shown in the following pseudo-code,
and generate the ACs to use the name to register or
lookup the service. For example, the Invoice’s
AC may comprise of the code to register its service
using the given name. Likewise, the Item’s AC
may looks-up for the services using the given name. |
|
Pseudo-code listing#10; Sample
Pseudo code for Directory services |
<svg
height="750" width="500">
<g transform="translate(450,10)">
<%
// Instantiate
& Initialize the CF.
AgileTemplate Invoice = new ShoppingCart(“ShoppingCart1”);
// Call the
method to generate the AC’s Code
Invoice.CGM(ati, out);
%>
</g>
<g transform="translate(10, 10)">
// Translate
(X, Y) determines the X & Y location.
<%
//Write
Java Code to use CF to include the AC’s code.
// Instantiate
& Initialize the CF
AgileTemplate Item = new ShoppingItemCar(Model,
“ShoppingCart1”);
// Call the
method to generate the AC’s Code
Item.CGM(ati, out);
%>
</g>
// Likewise,
many shopping items may be included in the web
page.
</svg> |
|
|
Pseudo-code listing#11; Pseudo
code for the Integration Logic |
<svg
height="750" width="500">
<g transform="translate(450,10)">
<%
// Instantiate
& Initialize the CF.
ShoppingCart Invoice = new ShoppingCart();
// Call the
method to generate the AC’s Code
Invoice.CGM(ati, out);
%>
</g>
<script> <![CDATA[
function add_item_to_the_invoice ( barcode,
Item_obj, xml_data) {
// Get the name
of Invoice’s Service method to add an Item. <%=Invoice.getAddItemMethod()%> (barcode,
Item_obj, xml_data);
}
]]>
</script>
<g transform="translate(10, 10)">
// Translate
(X, Y) determines the X & Y location.
<%
//Write Java Code
to use CF to include the AC’s code.
// Instantiate
& Initialize the CF
ShoppingItemCar Item1 = new ShoppingItemCar(Model);
// Register
the callback, which will be called upon selection
to buy.
Item1.addUpOnBuyCallback(“add_item_to_the_invoice”);
// Call the
method to generate the AC’s Code
Item1.CGM(ati, out);
%>
</g>
// Likewise, many
shopping items may be included in the web page.
</svg> |
|
|
Appendix-C::
Interfaces, an example for the data exchange format &
validation |
|
The
ACs usually communicate with each other by calling each
others functions (or methods) and exchange data through
the functions’ parameter and/or functions’
return objects. For successful completion of the communication,
the recipient of the message must know the format of
the message and able to get the minimum necessary data
to successfully complete the task. It is usually desirable
that many large ACs/CFs are designed and build independently
from each other. In such cases, the interfaces are predefined
and documented, so that, the ACs can be designed to
be compatible and properly exchange information after
the integration. |
|
For
example, a shopping cart application comprises of a
shopping-cart and many shopping items. The shopping
cart usually presented by an invoice-table, as shown
in the figure#5. It may support services, such as,
a function to add an item to the Invoice (i.e. add to
the shopping cart) and another function to delete an
item from the shopping-cart. |
|
When
customer selects an item’s AC to purchase the
item, the AC calls the service method to add the item
to the shopping-cart, and passes appropriate data, such
as, barcode or item-id, item’s name, make, model,
price, inventory and volume discounts. This information
may be passed through parameters, such as, data Objects
or in an XML-data string. |
|
The
format of the function parameters and data may be predefined
and documented at the beginning of the design of the
application, so that, each item’s AC can be built
to properly exchange data with the shopping-cart (or
AC for the Invoice). For example, if the shopping-cart’s
AC can accept an XML-string, then each item’s
AC may comprise of the XML-data string and pass the
reference to the string in one of the parameters to
the service function of the Invoice’s AC, to add
the Item to the Shopping-cart upon selection to purchase
the item. |
|
The
AC for each of the shopping-items may be integrated
with the AC for the Invoice to request its services.
For example, the application may comprise of the Integration-logic
code that gets the name of the service methods of the
AC for the shopping-cart. Then the integration logic
generates code for a callback, such that, its body comprises
of the code to set the parameters appropriately and
calls the shopping-cart’s service method. This
callback can be registered with each of the shopping-item. |
|
Fig#5 |
|
The
applications often need to be updated or add new features,
for example, over the time as the business needs change.
To accommodate this newer functionality, the components
(i.e. ACs) must be updated or replaced. Therefore, the
CF need to be redesigned or refined to update the AC.
When components are updated or replaced, proper care
must be taken in order to maintain the communication
interfaces it has with other components (i.e. ACs).
Some times it is required to update the other ACs to
become compatible with the updated interface. |
|
It
is desirable to have tools to detect the broken or mismatches
of the format of the data being exchanged between the
ACs, for example, when a component (i.e. AC) is replaced
or updated. In the preferred embodiment, the recipient
of the data may validate the data format before consuming
the data. To validate the data, the recipient may use
a data format that is designed to accommodate such validations.
Furthermore, the data format may also be extensible,
in order to adapt to evolving business needs. For example,
an XML-schema may be created to define the format of
the data to be exchanged. The XML-data exchanges between
the ACs may be validated against the said XML-schema. |
|
For
example, the service provider (i.e. AC) may define an
XML-schema, which defines vocabulary and pieces of data
needed to process the request for the service. The preferred
embodiment uses a tool to validate the compatibility
of the XML data messages exchanged between any two ACs..
This checking or validation of data messages’
compatibility helps detect some of the hard to find
integration errors. |
|
The
validation tool may be a generic tool that verifies
the compatibility of the XML-messages of the interacting
components. Each component may maintain private copy
and it would update the private XML-schema, when the
XML-message contents are updated. Each of the components
(i.e. AC) is designed to create (or consume) the XML-
data, which is conforms to its private copy. The consumer
component of the XML-message may request the tool to
validate the XML-data. Usually, the XML-data contains
a URI pointing to the private copy of the sender’s
XML-schema. |
|
For
example, the requesting AC may send the request message
(e.g. XML-data) to get a service. Before processing
the request, the service-provider AC may request the
XML-schema validation-tool to validate the XML-message
and requester’s XML-schemas against the service
provider’s XML-schema. If they are not compatible,
it may generate an error message that lists the incompatibilities.
This helps detect some of the data mismatch errors,
for example, if the ACs are inadvertently updated or
replaced. |
|
The
XML-schema usually defines the required data items and
optional data items in the XML-message. Hence, it is
not an error, if the optional data items are not included
in the XML-data string. To accommodate the newer functionality,
the service provider’s XML-schema may be updated.
If optional data fields added to the newer version of
the XML-schema, the service provider may continue to
serve the service requests conforming to the old version
of the XML-schema. If the recipient detects the mismatch
of the XML-formats but the missing fields are optional
data items, and if recipient can process the data without
the information, it may log warning messages and continue
servicing the requests. |
|
Likewise,
the requesting AC (i.e. consumer of the service) may
get an XML-data string in response to its request. It
may also validate the XML-data to detect a broken link
or potential data incompatibility. |
|
To
optimize the performance of the application, this validation
may be turned on only when the application is run in
testing mode. The validation-tool may comprise of a
flag, and supports a mechanism to turn on-or-off the
validation flag. If the flag is turned-off, it immediately
may returns with out performing any validation. |